Core API
Core settings and commands affect the entire widget.
Settings
The Web Widget (Classic) has the following core settings:
Example
<script type="text/javascript">
window.zESettings = {
webWidget: {
color: { theme: '#78a300' }
}
};
</script>
contactOptions
The widget's contactOptions
object, which represents a component that lets the user choose between starting a chat or submitting a ticket, has the following settings:
- enabled (Boolean)
- contactButton
- chatLabelOnline
- chatLabelOffline
- contactFormLabel
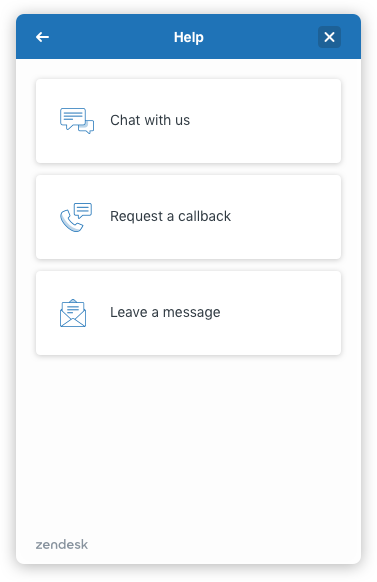
To learn more about contact options, see Offering end-users multiple contact options in the Zendesk help center.
Note: chatLabelOnline
and contactFormLabel
applies to the contact options shown to the end user on the Answer Bot channel.
Example
<script type="text/javascript">
window.zESettings = {
webWidget: {
contactOptions: {
enabled: true,
contactButton: { '*': 'Get in touch' }
}
}
};
</script>
launcher
The widget's launcher
object, which represents the launcher button, has the following settings:
- chatLabel
- label
- talkLabel
- mobile
- labelVisible
Example
<script type="text/javascript">
window.zESettings = {
webWidget: {
launcher: {
chatLabel: {
'*': 'Chat now'
},
mobile: {
labelVisible: true
}
}
}
};
</script>
Commands
The Web Widget (Classic) has the following core commands:
- clear
- close
- get display
- hide
- identify
- logout
- on open
- on close
- on userEvent
- open
- prefill
- reset
- setLocale
- show
- toggle
- updatePath
- updateSettings
clear
zE('webWidget', 'clear');
Clears all forms in the Web Widget.
Parameters
None
close
zE('webWidget', 'close');
If the widget is opened, this command closes the widget and shows the launcher.
Parameters
None
get display
zE('webWidget:get', 'display');
Gets the current widget display. Depending on the features you have enabled, the command displays one of the following strings: helpCenter
, chat
, contactForm
, talk
, contactOptions
, answerBot
, or hidden
.
hide
zE('webWidget', 'hide');
Hides all parts of the widget from the page. You can invoke it before or after page load.
Parameters
None
Example
Before page load:
<script type="text/javascript">
zE('webWidget', 'hide');
</script>
After page load:
<button onclick="zE('webWidget', 'hide')">Hide Web Widget</button>
identify
zE('webWidget', 'identify', data<object>);
Identifies an end user in the Chat dashboard and pre-fills the end-user's details in Chat forms inside the Web Widget.
Note:
- The Identify API only prepopulates the user's details in the Chat forms (Prechat, Chat Offline and Update Contact Details forms). To prefill all forms in any product configuration, use prefill.
Parameters
data
: Object. Contains the propertiesname
andemail
.
A console warning occurs when there are invalid keys, invalid data on valid keys, or when passing non-object types.
Example
<script type="text/javascript">
zE('webWidget', 'identify', {
name: 'Akira Kogane',
email: '[email protected]'
});
</script>
logout
zE('webWidget', 'logout');
Clears an end user's session.
Parameters
None
on open
zE('webWidget:on', 'open', callback<function>);
Executes a callback when the widget is opened.
Parameters
callback
: Function. Contains the code to be executed.
Example
<script type="text/javascript">
zE('webWidget:on', 'open', function() {
console.log('The widget has been opened!');
});
</script>
on close
zE('webWidget:on', 'close', callback<function>);
Executes a callback when the widget is closed.
Parameters
callback
: Function. Contains the code to be executed.
Example
<script type="text/javascript">
zE('webWidget:on', 'close', function() {
console.log('The widget has been closed!');
});
</script>
on userEvent
zE('webWidget:on', 'userEvent', function(userEvent<object>));
Executes a callback when a user event is fired. This can be used as a flexible way of integrating third party analytics tools into the widget, and filtering events sent to Google Analytics. This setting can be applied even when the analytics setting is used to disable user events tracking.
Parameters
callback
: Function. The callback to perform on each user event. Contains one parameter,userEvent
, an object that contains theaction
,properties
, andcategory
of the event.
properties
is either an object with the data for the given event or undefined
as shown below.
Widget
Action | Properties |
---|---|
Web Widget Opened | undefined |
Web Widget Minimised | undefined |
Chat
Action | Properties |
---|---|
Chat Opened | undefined |
Chat Shown | undefined |
Chat Served by Operator | { agent: <agent display name> } |
Chat Rating Good | undefined |
Chat Rating Bad | undefined |
Chat Rating Removed | undefined |
Chat Comment Submitted | undefined |
Chat Request Form Submitted | { department: <department name> }* |
* Department may be undefined.
Contact Form
Action | Properties |
---|---|
Contact Form Shown | { id: <form id>, name: <form name>}** |
Contact Form Submitted | { id: <form id>, name: <form name>}** |
** If ticket forms is not enabled, the property value is { name: 'contact-form'}
.
Help Center
Action | Properties |
---|---|
Help Center Shown | undefined |
Help Center Search | { term: <search term>} |
Help Center Article Viewed | { id: <article id>, name: <article name>} |
Help Center View Original Article Clicked | { id: <article id>, name: <article name>} |
Answer Bot
Note: Zendesk has renamed our bot capabilities. Answer Bot is now AI agents. Article Recommendations are now autoreplies.
Action | Properties |
---|---|
Answer Bot Article Viewed | { id: <article id>, name: <article name>} |
Talk
Action | Properties |
---|---|
Talk Shown | { contactOption: <talk contact option> } |
Talk Callback Request Submitted | undefined |
Example
<script type="text/javascript">
zE('webWidget:on', 'userEvent', function(event) {
console.log(event.category, event.action, event.properties);
});
</script>
open
zE('webWidget', 'open');
Forces the widget to open.
Parameters
None
prefill
zE('webWidget', 'prefill', data<object>);
Prefills an end user's details on the following forms: ticket, pre-chat, and chat offline. It does not prefill the callback form.
Parameters
data
: Object. Contains aname
,email
andphone
objects.
Example
<script type="text/javascript">
zE('webWidget', 'prefill', {
name: {
value: 'isamu',
readOnly: true // optional
},
email: {
value: '[email protected]',
readOnly: true // optional
},
phone: {
value: '61431909749',
readOnly: true // optional
}
});
</script>
reset
zE('webWidget', 'reset');
Completely resets the state of the widget. To preserve end-user experience, this API only functions when the widget is minimized.
Parameters
None
setLocale
zE('webWidget', 'setLocale', data<string>);
Sets the widget locale.
The command takes a locale string as an argument. For a list of supported locales and associated codes, see https://support.zendesk.com/api/v2/locales/public.json.
By default, the Web Widget (Classic) is displayed to the end user in a language that matches the browser header of their web browser. If you want to force the widget to be displayed in a specific language on your website, you can use zE('webWidget', 'setLocale', data<string>);
to specify the language.
Note: This code should be placed immediately after the Web Widget (Classic) code snippet.
Parameters
data
: String. The locale string to change the widget locale to.
Example
The following example displays the widget in German:
<script type="text/javascript">
zE('webWidget', 'setLocale', 'de');
</script>
show
zE('webWidget', 'show');
Displays the widget on the host page in the state it was in before it was hidden.
The widget is displayed by default on page load. You don't need to call show
to display the widget unless you use hide
.
Example
<script type="text/javascript">
zE('webWidget', 'show');
</script>
toggle
zE('webWidget', 'toggle');
Opens the widget if it was closed, or closes the widget if it was opened.
Parameters
None
updatePath
zE('webWidget', 'updatePath', data<object>);
Updates the visitor path by setting the title to the current user's page title and url to the user's current url.
Note: This API also updates the path within Chat.
Parameters
data
: Object. This object accepts two optional string parameterstitle
andurl
. Note that theurl
parameter must be a complete URL, including the scheme.
Example
<script type="text/javascript">
zE('webWidget', 'updatePath', {
title: 'Voltron',
url: 'https://example.com/voltron'
});
</script>
updateSettings
zE('webWidget', 'updateSettings', data<object>);
Updates the widget's zESettings. It can update multiple settings at once.
Parameters
data
: Object. Matches the structure defined in zESettings
Example
<script type="text/javascript">
zE('webWidget', 'updateSettings', {
webWidget: {
chat: {
departments: {
enabled: ['finance', 'hr', 'sales'],
select: 'sales'
}
}
}
});
</script>