Building your first Sell app - Part 2: Designing the user interface
This tutorial is the second part of a series on building a Zendesk Sell app:
- Part 1: Laying the groundwork
- Part 2 - Designing the user interface - YOU ARE HERE
- Part 3: Creating and inserting templates
- Part 4: Getting data
- Part 5: Installing the app in Zendesk Sell
In the previous tutorial, you installed ZCLI and created starter files for an app named Related Leads. In this tutorial, you'll work on the app's user interface (UI). The tutorial covers the following tasks:
- Setting the app's location in Sell
- Reviewing the main HTML file
- Testing the app
- Cleaning up the default code
- Adding a footer
- Adding styles
- Changing the logo
Setting the app's location in Sell
You can run an app in different locations of the Sell UI. This app will display in the sidebar of the deal card:
To set the app location in Sell
-
In a text editor, open the file named manifest.json in your app folder (/related_leads/manifest.json).
-
Replace the default
location
property with the following:"location": {
"sell": {
"deal_card": "assets/iframe.html"
}
},
-
Save manifest.json.
Reviewing the main HTML file
Your app will display in an iframe in the deal_card
location you specified.
In the previous tutorial, ZCLI created an iframe.html file in your app's assets folder (related_leads/assets/iframe.html). Open the iframe.html file in your text editor.
To interact with the Zendesk Apps framework (ZAF), the HTML file imports the ZAF SDK library in a script tag:
<script src="https://static.zdassets.com/zendesk_app_framework_sdk/2.0/zaf_sdk.min.js"></script>
After importing the SDK, you can use the ZAFClient.init()
method to create a ZAF API Client:
var client = ZAFClient.init();
The ZAF Client provides an interface between your app and a host application, such as Zendesk Sell. The client provides methods you can use to interact with ZAF. For example, the starter file includes:
client.invoke('resize', { width: '100%', height: '200px' });
Testing the app
Your app doesn't do much yet, but you can already run it using ZCLI. Run your app often to test your latest changes.
Tip: Use private windows or the Incognito mode in your browser when testing and developing apps. Your browser may cache certain files used by the app. If a change is not working in your app, the browser might be using an older cached version of the file. With private browsing, files aren't cached.
To test your app
-
In your command-line interface, navigate to the related_leads folder. For example:
$ cd projects/related_leads
-
Start a local ZCLI web server by running the following command:
$ zcli apps:server
After a moment, a status message appears informing you that the server has started.
-
In your browser's private or incognito window, sign in to Sell.
-
Go to the Deals page and select a deal from the list to open a deal card. The URL should look like this:
https://app.futuresimple.com/sales/deals/12345678
-
Append ?zcli_apps=true to the deal card URL and press Enter. The URL should look like this:
https://app.futuresimple.com/sales/deals/12345678?zcli_apps=true
Tip: Bookmark the modified URL for easy access in the future.
In the sidebar, the app displays a "Hello, World!" heading. This heading is specified in the app's iframe.html file.
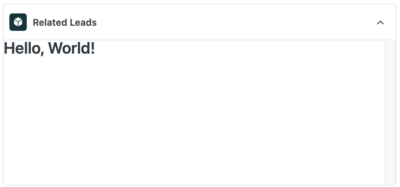
If you compare the current layout of the app against your earlier mockup, there are still some changes to make:
- Clean up the default code. This includes replacing the "Hello, World!" heading, adding a list for leads, and moving the JavaScript in iframe.html to another file.
- Add a footer that displays "Report bugs" with a link to a website where users can report bugs.
- Add styles to format the app's UI
- Replace the default logo in the header
Cleaning up the default code
You can start by cleaning up the default code in iframe.html.
-
Open iframe.html in a text editor and replace the
<h2>
tag with the following HTML:<h2>New leads for the organization</h2>
<ul>
<li><a href="" target="_blank">Lead one</a></li>
<li><a href="" target="_blank">Lead two</a></li>
</ul>
For now, the HTML contains placeholder values. It's okay that these values don't match the mockup yet. You'll update the app to use live data in a later tutorial.
-
Create a file named main.js in the assets folder. Resources such as HTML, JavaScript, CSS, and image files must be located in the assets folder.
-
Add the following function to main.js:
(function () {
const client = ZAFClient.init();
client.invoke("resize", { width: "100%", height: "150px" });
})();
The
(function () {...})();
statement defines an anonymous, self-invoking function. The function runs after the document is loaded in the browser. The function's two statements are similar to the ones contained in the<script>
tag in iframe.html. -
In iframe.html, delete the following
<script>
tag:<script>
// Initialise Apps framework client. See also:
// https://developer.zendesk.com/apps/docs/developer-guide/getting_started
var client = ZAFClient.init();
client.invoke('resize', { width: '100%', height: '200px' });
</script>
-
Replace it with the following
<script>
tag to import the main.js file:<script src="main.js"></script>
Insert the tag after the
<script>
tag that references the ZAF SDK. This ensures the SDK loads before main.js runs.The
<body>
tag of iframe.html should now look like this:<body>
<h2>New leads for the organization</h2>
<ul>
<li><a href="" target="_blank">Lead one</a></li>
<li><a href="" target="_blank">Lead two</a></li>
</ul>
<script src="https://static.zdassets.com/zendesk_app_framework_sdk/2.0/zaf_sdk.min.js"></script>
<script src="main.js"></script>
</body>
-
Save iframe.html and main.js. To review your changes, click the refresh icon above your app.
Adding a footer
According to the mockup, the footer should display a Report bugs link. This link lets users report bugs for the app.
To add a footer link
-
In the iframe.html file, add the following footer tag before the script tags:
<footer>
<a href="https://example.com/support" target="_blank">Report bugs</a>
</footer>
-
Save iframe.html and click the refresh icon.
In the mockup, the footer text is smaller. In the next step, you'll add some CSS to style the footer and other parts of the app UI.
Adding styles
By default, ZCLI imports a Zendesk Garden stylesheet in iframe.html:
<link rel="stylesheet" href="https://cdn.jsdelivr.net/combine/npm/@zendeskgarden/[email protected],npm/@zendeskgarden/[email protected]">
Zendesk Garden is designed to be a common baseline of styles and components between all Zendesk products. The default link above doesn't include all Zendesk Garden assets, only the Garden component CSS and CSS utilities. A complete list of assets you can import is available from the jsDelivr CDN. See https://www.jsdelivr.com/?query=zendeskgarden. The jsDelivr files are npm packages that can also be installed from npm. See https://www.npmjs.com/search?q=zendeskgarden.
Zendesk recommends integrating the individual CSS files currently hosted on jsDelivr into your application files and referencing them using relative paths within your application. This helps prevent connectivity issues by hosting your assets on Zendesk's own app CDN and not a third-party CDN.
Disclaimer: Zendesk is not able to provide support for third party package hosting services, such as NPM or JsDelivr. Consider posting the issue to our developer community or searching online.
Use the Zendesk Garden assets if you want your app to match the look and feel of Zendesk products. However, apps aren't required to use Zendesk Garden. The choice is yours.
This app will use the default Zendesk Garden styles and add modifications in a separate stylesheet named main.css.
-
In iframe.html, add the following
<link>
tag after the existing Zendesk Garden stylesheet:<link rel="stylesheet" href="main.css">
-
Create a file named main.css in the assets folder.
-
Add the following rules to main.css :
body {
margin-top: 10px;
padding-left: 40px;
}
h2 {
margin-bottom: 10px;
font-weight: 600;
}
ul {
list-style-type: disc;
margin-left: 25px;
}
footer {
font-size: 10px;
margin-top: 20px;
}
-
Save iframe.html and main.css. Then click the refresh icon.
Changing the logo
By default, the framework displays an image named logo-small.png in your assets folder. To change the logo of the app, replace the logo-small.png file in the assets folder with your own image.
Your replacement logo-small.png file should meet the following specs:
- Size: 128x128 pixels. The CSS will scale the image to fit the header. The same image will also be used in the Zendesk Sell UI later and scaled accordingly.
- File format: PNG-24 with transparency.
- Border: None. The corners will be rounded by the CSS.
The assets folder also includes a logo.png image. Replace this image with a 320x320 version of your logo. The Zendesk Marketplace will display this version of the logo. Sell admins can use the Zendesk Marketplace to browse for public or private apps to install.
Change the logo
-
Right-click each of the following image links and download them into the assets folder in your app folder. If prompted, agree to replace the existing version of the files.
-
Click the refresh icon.
If the updated image doesn't appear, you'll need to do a hard refresh of the page. See the instructions for Firefox or Chrome on their respective websites. You can also paste the page URL into another browser.
If you're moving to the next tutorial, you can leave the ZCLI server running. If you're done for this session, you can shut down the server by switching to your command-line interface and pressing Ctrl+C.
In the next tutorial, you'll create templates to display leads and other data in the app. Continue to Part 3: Creating and inserting templates.