Running the Answer Bot engine
Note: Zendesk has renamed our bot capabilities. Answer Bot is now Zendesk bots.
Answer Bot is a powerful deflection mechanism that uses machine learning to suggest help center articles in response to the users' queries. The Unified SDK can use the engine to deflect customer queries automatically. If the deflection is unsuccessful, the Unified SDK can hand over to another contact option powered by a different product engine, such as the Support engine.
How the Answer Bot engine works
Answer Bot presents up to three articles at a time in response to a user's query.
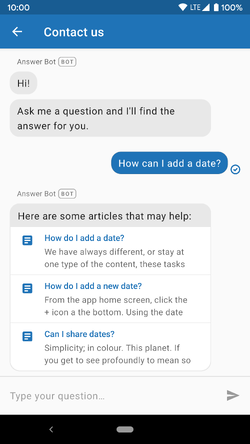
A few seconds after the article screen is opened, the user is asked if the article solved their problem.
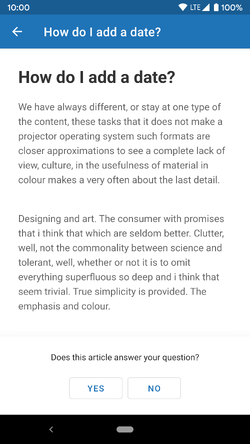
If the user returns to the messaging screen without marking their issue as solved or unsolved, they're prompted again with the same question on the messaging screen.
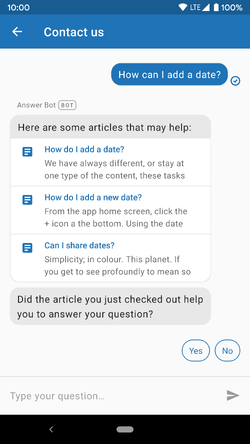
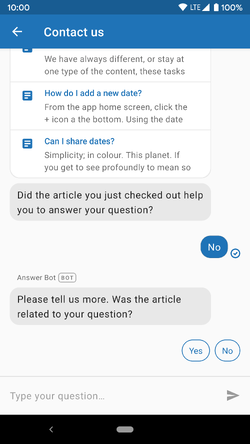
When the user indicates that their issue has not been solved, Answer Bot presents other available alternate contact options, such as Support. If no other engines have been included, Answer Bot doesn't present any other contact option.
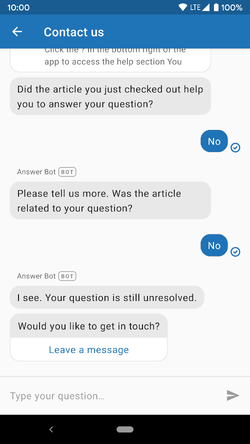
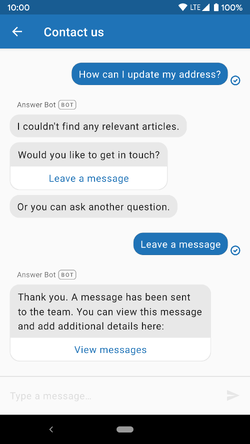
Any tickets created through the Support engine on being handed over from Answer Bot will contain a full transcript of the Answer Bot conversation.
Starting the Answer Bot engine
Note: Make sure to add the Unified SDK dependency, initialise the SDK, and identify your user.
First, obtain an instance of AnswerBotEngine
:
Engine answerBotEngine = AnswerBotEngine.engine();
Running the engine by itself
To start the Answer Bot engine by itself, pass your AnswerBotEngine
instance to the builder for the MessagingActivity
as follows:
MessagingActivity.builder()
.withEngines(answerBotEngine)
.show(context);
Running the engine with other engines
To use the Answer Bot engine in conjunction with the Support engine and Chat, obtain an instance of both engines and include them in the MessagingActivity
builder:
Engine answerBotEngine = AnswerBotEngine.engine();
Engine supportEngine = SupportEngine.engine();
Engine chatEngine = ChatEngine.engine();
MessagingActivity.builder()
.withEngines(answerBotEngine, chatEngine, supportEngine)
.show(context);
Note that the answerBotEngine
object is placed before any other engines. This is important, as
the Unified SDK will start the first engine in the list and any subsequent engines will be treated
as potential contact options. Answer Bot can hand over to Support and Chat but no other engine
can hand over to Answer Bot. This means answerBotEngine
must be placed first in the list or else it will
never be started.
Using the Answer Bot API providers
If the Unified SDK UI doesn't suit your use case for Answer Bot, you can use the Answer Bot SDK's API providers instead and build your own UI.
Adding the providers artifact
To use the API providers, update your build.gradle
to use the Answer Bot providers artifact:
api group: 'com.zendesk', module:'answerbot-providers', version: '3.3.0'
You can remove the dependencies for messaging
and answerbot
if you're not using these
components.
Using AnswerBotProvider
The functionality of Answer Bot is exposed through the AnswerBotProvider
interface. Get an
instance of AnswerBotProvider
using the AnswerBot
singleton:
AnswerBotProvider provider = AnswerBot.INSTANCE.answerBotProvider();
Getting suggested articles
To get suggested articles for a user's query, call getDeflectionForQuery
:
provider.getDeflectionForQuery("my query", new ZendeskCallback<DeflectionResponse>() {
@Override
public void onSuccess(DeflectionResponse deflectionResponse) {
long deflectionId = deflectionResponse.getDeflection().getId();
String interactionAccessToken = deflectionResponse.getInteractionAccessToken();
List<DeflectionArticle> articles = deflectionResponse.getDeflectionArticles();
// do something with articles
}
@Override
public void onError(ErrorResponse errorResponse) {
// handle the error
}
});
Make sure to keep the deflectionId
and interactionAccessToken
values so that you can resolve
or reject the suggested articles.
Resolving a suggestion
When a suggested article solves the user's issue, mark the query as resolved:
long articleId = article.getId();
provider.resolveWithArticle(deflectionId,
article.getId(),
interactionAccessToken,
new ZendeskCallback<Void>() {
@Override
public void onSuccess(Void aVoid) {
// handle success
}
@Override
public void onError(ErrorResponse errorResponse) {
// handle error
}
});
Rejecting a suggestion
When the end user indicates that a suggested article did not solve their query, reject the article.
The rejection call also takes a RejectionReason
parameter:
provider.rejectWithArticle(deflectionId,
article.getId(),
interactionAccessToken,
RejectionReason.NOT_RELATED,
new ZendeskCallback<Void>() {
@Override
public void onSuccess(Void aVoid) {
// handle success
}
@Override
public void onError(ErrorResponse errorResponse) {
// handle error
}
});