Running the Answer Bot engine
Running the Answer Bot engine
Answer Bot is a powerful deflection mechanism that uses machine learning to suggest help center articles in response to the users' queries. The Unified SDK can use the engine to deflect customer queries automatically. If the deflection is unsuccessful, the Unified SDK can hand over to another contact option powered by a different product engine, such as the Support engine.
How the Answer Bot engine works
Answer Bot presents up to three articles at a time in response to a user's query.
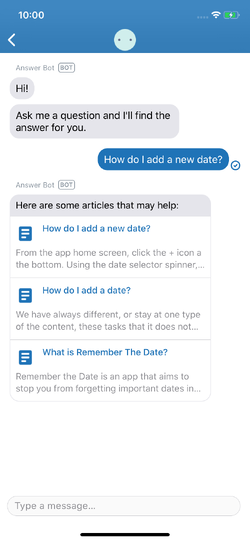
A few seconds after the article screen is opened, the user is asked if the article solved their problem.
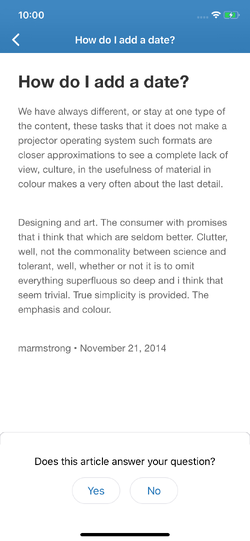
If the user returns to the messaging screen without marking their issue as solved or unsolved, they're prompted again with the same question on the messaging screen.
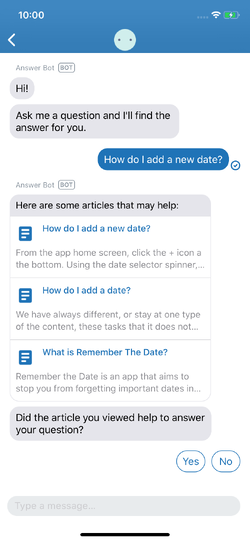
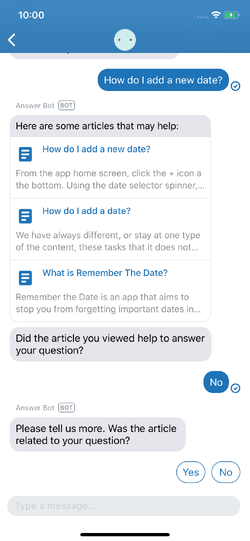
When the user indicates that their issue has not been solved, Answer Bot presents other available alternate contact options, such as Support. If no other engines have been included, Answer Bot doesn't present any other contact option.
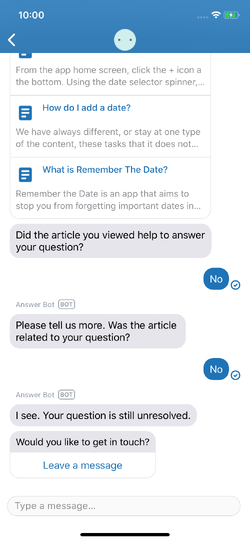
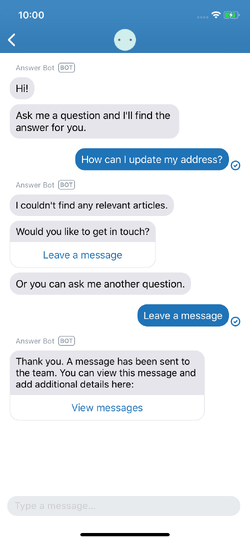
Any tickets created through the Support engine on being handed over from Answer Bot will contain a full transcript of the Answer Bot conversation.
Starting the Answer Bot engine
Note: Make sure to add the Unified SDK dependency, initialise the SDK, and identify your user.
First, import the necessary dependencies:
Swift
import AnswerBotSDK
import MessagingSDK
import MessagingAPI
import SDKConfigurations
Objective-C
#import <MessagingSDK/MessagingSDK.h>
#import <AnswerBotSDK/AnswerBotSDK.h>
#import <MessagingAPI/MessagingAPI.h>
#import <SDKConfigurations/SDKConfigurations.h>
Second, get an instance of an AnswerBotEngine
engine:
Swift
do {
let answerBotEngine = try AnswerBotEngine.engine()
} catch {
// do something with error
}
Objective-C
NSError *error = nil;
ZDKAnswerBotEngine *answerBotEngine = [ZDKAnswerBotEngine engineAndReturnError:&error];
Running the engine by itself
To start the Answer Bot engine by itself on the Messaging screen, pass your AnswerBotEngine
instance to the buildUI
method on Messaging
to get an instance of an UIViewController
:
Swift
do {
let messagingConfiguration = MessagingConfiguration()
let viewController = try Messaging.instance.buildUI(engines: [answerBotEngine],
configs: [messagingConfiguration])
self.navigationController?.pushViewController(viewController, animated: true)
} catch {
// do something with error
}
Objective-C
- (void) startConversation {
NSError *error = nil;
NSArray *engines = @[ (id <ZDKEngine>) [ZDKAnswerBotEngine engineAndReturnError:&error] ];
ZDKClassicMessagingConfiguration *messagingConfiguration = [ZDKClassicMessagingConfiguration new];
UIViewController *viewController = [[ZDKClassicMessaging instance] buildUIWithEngines:engines
configs:@[messagingConfiguration]
error:&error];
[self.navigationController pushViewController:viewController animated:YES];
}
Running the engine with other engines
To use the Answer Bot engine in conjunction with the Support and Chat engines, obtain an instance of the desired engines and pass them with the buildUI
method:
Swift
do {
let messagingConfiguration = MessagingConfiguration()
let answerBotEngine = try AnswerBotEngine.engine()
let supportEngine = try SupportEngine.engine()
let chatEngine = try ChatEngine.engine()
let viewController = try Messaging.instance.buildUI(engines: [answerBotEngine, chatEngine, supportEngine],
configs: [messagingConfiguration])
self.navigationController?.pushViewController(viewController, animated: true)
} catch {
// do something with error
}
Objective-C
- (void) startConversation {
NSError *error = nil;
NSArray *engines = @[
(id <ZDKEngine>) [ZDKAnswerBotEngine engineAndReturnError:&error],
(id <ZDKEngine>) [ZDKSupportEngine engineAndReturnError:&error],
(id <ZDKEngine>) [ZDKChatEngine engineAndReturnError:&error],
];
ZDKClassicMessagingConfiguration *messagingConfiguration = [ZDKClassicMessagingConfiguration new];
UIViewController *viewController = [[ZDKClassicMessaging instance] buildUIWithEngines:engines
configs:@[messagingConfiguration]
error:&error];
[self.navigationController pushViewController:viewController animated:YES];
}
Note that the answerBotEngine
object is placed before any other engines. This is important because
the Unified SDK will start the first engine in the list and any subsequent engines will be treated
as potential contact options. Answer Bot can hand over to Support and Chat, but no other engine
can hand over to Answer Bot. This means answerBotEngine
must be placed first in the list or else it will
never be started.
Using the Answer Bot API providers
If the Unified SDK UI doesn't suit your use case for Answer Bot, you can use the Answer Bot SDK's API providers instead and build your own UI.
Adding the providers as a dependency
To add only the providers as a dependency, see Add SDK.
Using AnswerBotProvider
Get an instance of AnswerBotProvider
using the AnswerBot
singleton:
Swift
let answerBotProvider = AnswerBot.instance?.provider
Objective-C
ZDKAnswerBotProvider* provider = [[ZDKAnswerBot instance] provider];
Getting suggested articles
To get suggested articles for a user's query, call getDeflectionForQuery
:
Swift
answerBotProvider.getDeflectionForQuery(query: "query") { (result) in
switch result {
case .success(let deflectionResponse):
let deflectionId = deflectionResponse.deflectionId
let interactionAccessToken = deflectionResponse.interactionAccessToken
// do something with articles
case .failure:
// handle error
}
}
Objective-C
[provider getDeflectionForQueryWithQuery:@"query" callback:^(ZDKDeflectionResponse * _Nullable response, NSError * _Nullable error) {
NSNumber *deflectionId = [NSNumber numberWithUnsignedLong:response.deflection.deflectionID];
NSString *interactionAccessToken = response.interactionAccessToken;
NSArray<ZDKDeflectionArticle *>* articles = response.deflectionArticles;
// do something with articles
}];
Make sure to keep the deflectionId
and interactionAccessToken
values so you can resolve
or reject the suggested articles.
Resolving a suggestion
When a suggested article solves the user's issue, mark the query as resolved:
Swift
answerBotProvider.resolveWithArticle(deflectionId: deflectionId,
articleId: article.id,
interactionAccessToken: interactionAccessToken) { (result) in
switch result {
case .success:
// handle success
case .failure:
// handle error
}
}
Objective-C
[provider resolveWithArticleWithDeflectionId:deflectionId
articleId:article.id
interactionAccessToken: interactionAccessToken
callback:^(id _Nullable result, NSError * _Nullable error) {
// handle outcome
}];
Rejecting a suggestion
When the end user indicates that a suggested article did not solve their query, reject the article.
The rejection call also takes a RejectionReason
parameter:
Swift
answerBotProvider.rejectWithArticle(deflectionId: delfactionId,
articleId: article.id,
interactionAccessToken: interactionAccessToken,
reason: .notRelated) { (result) in
switch result {
case .success(let response):
// do something with success
case .failure:
// do something with error
}
}
Objective-C
[provider rejectWithArticleWithDeflectionId:deflectionid
articleId:article.id
interactionAccessToken:InteractionAccessToken
reason:ZDKRejectionReasonNotRelated
callback:^(id _Nullable result, NSError * _Nullable error) {
// handle outcome
}];