Adding your help center
The help center by Zendesk Guide provides your customers with a web-based, self-service knowledge base. You can use the Support SDK to show localized content in your help center, filter its contents to be more helpful, and use it as a jumping off point for creating a ticket.
The HelpCenterActivity adds your help center to your Android app.
If you need to build your own UIs, the SDK also has an API provider that gives you access to your organization's help center. See HelpCenterProvider in the Support SDK Javadocs.
Topics covered in this section:
- Prerequisites
- UI overview
- Show your help center
- Filter articles by category
- Filter articles by section
- Filter articles by label
- Show a single article
- Configure the ticket form
- Disable ticket creation
- Disable article voting
- Using Support SDK with Answer Bot SDK
- Overriding device locale
Prerequisites
-
A Zendesk admin has activated the help center in Zendesk Support. See Activating your help center in Zendesk help
-
One or more articles have been published in the knowledge base
-
A Zendesk admin has enabled Enable Guide for your Android app in Zendesk Support. See Configuring the SDK in Zendesk Support in Zendesk help
-
You have a theme in your Android app which inherits from
Theme.MaterialComponents
. If inheriting fromTheme.AppCompat
, some of the UI elements in HelpCenterActivity will display incorrectly. See Customizing the look for more information.
UI overview
The default UI of the HelpCenterActivity looks as follows:
To change the look and feel of the UI, see Customizing the look. The UI doesn't inherit any styling from the web-based version of your help center.
The UI is localized in 33 languages and selects the appropriate language based on the device locale. The locale must exist in your help center and in your Support account localization settings. The articles in your help center must be localized to view them in another language.
Tapping an article in the list shows the article content:
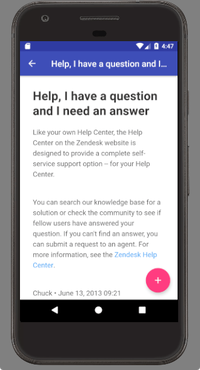
If the user can't find an answer in the help center, they have the option of tapping a Plus (+) button to open a ticket form:
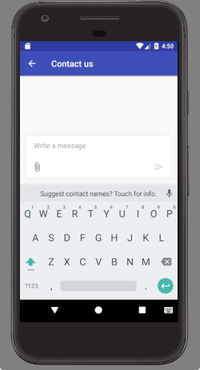
To configure the activity's ticket form, see Configure the ticket form of other SDK activities.
You can disable the ticket form if you don't want it, or if you prefer adding a separate ticket form.
Show your help center
Use the show()
method of the HelpCenterActivity builder. Example:
HelpCenterActivity.builder()
.show(MyActivity.this);
See show in HelpCenterActivity builder.
Note: Above a certain number of articles, the SDK starts to limit how many are fetched and displayed out of performance reasons. If this occurs, the recommended approach is to use the various filters on categories, sections, or labels that we provide to limit and control the amount of results.
See Known issues.
Filter articles by category
Use the withArticlesForCategoryIds()
method of the HelpCenterActivity builder. Example:
HelpCenterActivity.builder()
.withArticlesForCategoryIds(112233L, 223344L)
.show(this);
See withArticlesForCategoryIds in HelpCenterActivity builder.
Filter articles by section
Use the withArticlesForSectionIds()
method of the HelpCenterActivity builder. Example:
HelpCenterActivity.builder()
.withArticlesForSectionIds(112233L, 223344L)
.show(this);
See withArticlesForSectionIds in HelpCenterActivity builder.
Filter articles by label
Use the withLabelNames()
method of the HelpCenterActivity builder. Example:
HelpCenterActivity.builder()
.withLabelNames("android", "android_o")
.show(this);
See withLabelNames in HelpCenterActivity builder.
Show a single article
To open a specific article, get the article's id, then start the ViewArticleActivity
with its builder.
ViewArticleActivity.builder(123L)
.show(this);
See ViewArticleActivity builder.
Configure the ticket form
You can configure the ticket form of the HelpCenterActivity so that each new ticket has a common subject, common tags, or common file attachments. You can also set common values for one or more custom ticket fields, or set the custom ticket form to use agent interface in the Zendesk Support.
First, configure the ticket form with the config()
method of the RequestActivity builder. See config in the RequestActivity reference. The config()
method returns a Configuration object.
Second, pass the Configuration object to the show
method of the HelpCenterActivity builder.
Example:
Configuration requestActivityConfig = RequestActivity.builder()
.withRequestSubject("Android ticket")
.withTags("android", "mobile")
.config();
HelpCenterActivity.builder()
.show(MyActivity.this, requestActivityConfig);
See config in RequestActivity builder.
Disable ticket creation
The help center has floating action buttons that the user can tap to open a UI to create a ticket. The button is visible by default in both the help center article list view and article view. You can hide the button in one or both places.
Disable ticket creation in the article list view
Use the withContactUsButtonVisible()
and the withShowConversationsMenuButtonEnabled()
methods of the HelpCenterActivity builder. Example:
HelpCenterActivity.builder()
.withContactUsButtonVisible(false)
.withShowConversationsMenuButton(false)
.show(this);
See withContactUsButtonVisible in HelpCenterActivity builder.
Disable ticket creation in the article view
Create a config object, then pass it to HelpCenterActivity.
Configuration articleConfig = ViewArticleActivity.builder()
.withContactUsButtonVisible(false)
.config();
HelpCenterActivity.builder()
.show(this, articleConfig);
See withContactUsButtonVisible and config in ViewArticleActivity builder.
Disable ticket creation in both views
Combine the withContactUsButtonVisible()
method with a Configuration object from the ViewArticleActivity:
Configuration articleConfig = ViewArticleActivity.builder()
.withContactUsButtonVisible(false)
.config();
HelpCenterActivity.builder()
.withContactUsButtonVisible(false)
.show(this, articleConfig);
See withContactUsButtonVisible and config in ViewArticleActivity builder.
Disable article voting
The help center article voting feature in the Support SDK can be enabled and disabled by a Zendesk admin in Zendesk Support. It is enabled by default. See Configuring the SDK in Zendesk Support in Zendesk help.
This article voting is entirely separate to resolutions and rejections in Answer Bot.
Using Support SDK with Answer Bot SDK
Note: Zendesk has renamed our bot capabilities. Answer Bot is now Zendesk bots. For more information on this change, see this announcement.
Answer Bot by Zendesk provides a powerful mechanism for deflecting tickets before they reach your agents. The mobile SDK for Answer Bot is currently in an Early Access Program.
If you have Answer Bot 1.0.0
and Support SDK 2.3.0 (or above) present in your app, then by default the contact button in HelpCenterActivity
and ViewArticleActivity
opens Answer Bot. You can disable this behavior in both activities and direct the user straight to ticket creation.
To disable the deflection to Answer Bot in the help center article list view:
HelpCenterActivity.builder()
.withDeflectionEnabled(false)
.config();
To disable it in the help center article view:
ViewArticleActivity.builder()
.withDeflectionEnabled(false)
.config()
If you want to disable it in both screens, provide both configurations when starting the SDK.
Overriding device locale
If you want to manually specify what language to use when fetching help center content, you can do so as follows:
Support.INSTANCE.setHelpCenterLocaleOverride(locale);
This applies to all help center content fetched with the UI and providers, meaning that only the articles, sections, and categories from this locale are fetched. This does not update the mobile UI elements.
The override ignores the device locale and the languages configured in the Zendesk Guide admin console. Overriding with
an unsupported Locale
or no content for that locale results in a "Unable to connect" banner.