Running the Chat engine
Running the Chat engine
How the Chat engine works
The Chat engine offers a live chat experience between an agent and a customer. On startup it looks to see if a chat is ongoing and opens the chat if present. The engine has configuration options to enable and disable features like the pre-chat form for collecting visitor info. It also as an option to send and receive offline messages if no agents are online.
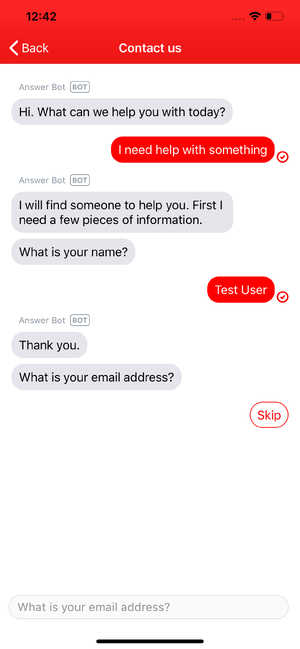
The engine presents this in a seamless conversational format. It sits on top of our Unified SDK, which supports handovers from the AnswerBot engine.
Starting the Chat engine
Note: Make sure to add the Unified SDK dependency, initialise the SDK, and identify your user.
First, import the necessary dependencies:
Swift
import ChatSDK
import ChatProvidersSDK
import MessagingSDK
import MessagingAPI
import SDKConfigurations
Objective-C
#import <ChatSDK/ChatSDK.h>
#import <ChatProvidersSDK/ChatProvidersSDK.h>
#import <MessagingSDK/MessagingSDK.h>
#import <MessagingAPI/MessagingAPI.h>
#import <SDKConfigurations/SDKConfigurations.h>
Second, get an instance of an ChatEngine
engine:
Swift
do {
let chatEngine = try ChatEngine.engine()
} catch {
// handle error
}
Objective-C
NSError *error = nil;
ZDKChatEngine *chatEngine = [ChatEngine engineAndReturnError:&error];
Running the engine by itself
To start the Chat engine by itself on the Messaging screen, pass your ChatEngine instance to the buildUI
method on Messaging
to get an instance of an UIViewController
:
Swift
func startConversation() throws {
let messagingConfiguration = MessagingConfiguration()
let chatEngine = try ChatEngine.engine()
let viewController = try Messaging.instance.buildUI(engines: [chatEngine],
configs: [messagingConfiguration])
self.navigationController?.pushViewController(viewController, animated: true)
}
Objective-C
- (void) startConversation {
NSError *error = nil;
NSArray *engines = @[ (id <ZDKEngine>) [ZDKChatEngine engineAndReturnError:&error] ];
ZDKClassicMessagingConfiguration *messagingConfiguration = [ZDKClassicMessagingConfiguration new];
UIViewController *viewController = [[ZDKClassicMessaging instance] buildUIWithEngines:engines
configs:@[messagingConfiguration]
error:&error];
[self.navigationController pushViewController:viewController animated:YES];
}
Running the engine with other engines
To use the Chat engine in conjunction with the AnswerBot and theSupport engine, obtain an instance of the desired engines and pass them with the buildUI
method:
Swift
func startConversation() throws {
let messagingConfiguration = MessagingConfiguration()
let answerBotEngine = try AnswerBotEngine.engine()
let supportEngine = try SupportEngine.engine()
let chatEngine = try ChatEngine.engine()
let viewController = try Messaging.instance.buildUI(engines: [answerBotEngine, chatEngine, supportEngine],
configs: [messagingConfiguration])
self.navigationController?.pushViewController(viewController, animated: true)
}
Objective-C
- (void) startConversation {
NSError *error = nil;
NSArray *engines = @[
(id <ZDKEngine>) [ZDKAnswerBotEngine engineAndReturnError:&error],
(id <ZDKEngine>) [ZDKSupportEngine engineAndReturnError:&error],
(id <ZDKEngine>) [ZDKChatEngine engineAndReturnError:&error],
];
ZDKClassicMessagingConfiguration *messagingConfiguration = [ZDKClassicMessagingConfiguration new];
UIViewController *viewController = [[ZDKClassicMessaging instance] buildUIWithEngines:engines
configs:@[messagingConfiguration]
error:&error];
[self.navigationController pushViewController:viewController animated:YES];
}
Note that the answerBotEngine
object is placed before any other engines. This is important, as
Unified SDK will start the first engine in the list, and any subsequent engines will be treated
as potential contact options. Answer Bot can hand over to Support and Chat but no other engine
can hand over to Answer Bot. This means answerBotEngine
must be placed first in the list or else it will
never be started.
Using the Chat API providers
See Providers for an in-depth look at the Chat API providers.