Adding Tickets
Adding Tickets
The conversations your organization has with customers appear as tickets in Zendesk Support. The Support SDK allows customers to use your app to create tickets, update existing ones, or see when their tickets have been updated by the customer service team.
All four Support SDK controllers, including the help center controllers, let the user create tickets. The following controllers also let the user view and update one or more of their tickets, and give you more control over tickets in your app:
- RequestController
- RequestListController
Note: Tickets in Zendesk are also known as support requests, or simply requests.
The RequestController lets you:
- Show and configure a ticket screen that a user can use to submit tickets to your customer service team
- Create a
UIViewController
to show the ticket screen later - Show one of the user's tickets
- Configure the ticket screen of other SDK controllers
The RequestListController lets you show a list of the user's tickets. The user can review and update their tickets.
If you need to build your own ticket UIs, the SDK also has an API provider that gives you access to tickets in Zendesk Support. See Request provider in the API providers reference.
Topics covered in this section:
- Prerequisites
- UI overview
- Show a ticket screen
- Configure a ticket screen
- Configure the ticket screen of other SDK controllers
- Show the user's tickets
- Show an existing ticket
- Check for ticket updates
Prerequisites
There is no prerequisite for letting users create tickets.
The following prerequisite must be met to let users view and update their tickets:
- A Zendesk admin has enabled Conversations for your app in Zendesk Support. See Configuring the SDK in Zendesk Support in Zendesk help
UI overview
The default ticket form of the RequestController looks as follows:
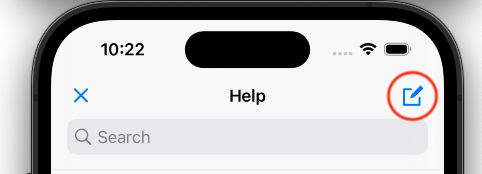
The default ticket list of the RequestListController looks as follows:
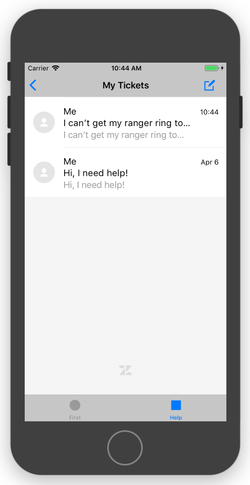
A bullet appears next to a ticket to alert the user when an agent has updated the ticket.
Tapping a ticket in the list shows the ticket conversation. The user can add a comment to the conversation.
You can change the look and feel of the UIs. See Customize the look.
Show a ticket screen
Use the buildRequestUi(with configurations: [ZDKConfiguration])
method of the RequestUi. Example:
Swift
let requestScreen = RequestUi.buildRequestUi(with: [])
self.navigationController?.pushViewController(requestScreen, animated: true)
Objective-C
UIViewController *requestScreen = [ZDKRequestUi buildRequestUiWith:@[]];
[self.navigationController pushViewController:requestScreen animated:YES];
If you prefer, you can use the ticket screen in the Request List Screen, Help Center Screen, or Article Screen. The UI of each controller has a button that lets the user open the ticket form.
Configure a ticket screen
Use the RequestUiConfiguration
class to configure the screen and tickets created from it.
Example:
Swift
let customFieldOne = CustomField(fieldId: 1234567, value: "some_value")
let config = RequestUiConfiguration()
config.subject = "iOS Ticket"
config.tags = ["ios", "mobile"]
config.customFields = [customFieldOne]
let requestController = RequestUi.buildRequestUi(with: [config])
self.navigationController?.pushViewController(requestController, animated: true)
Objective-C
ZDKCustomField *customFieldOne = [[ZDKCustomField alloc] initWithFieldId:@1234567 value:@"some_value"];
ZDKRequestUiConfiguration * config = [ZDKRequestUiConfiguration new];
config.subject = "iOS Ticket"
config.tags = ["ios", "mobile"]
config.customFields = [customFieldOne]
UIViewController *requestController = [ZDKRequestUi buildRequestUiWith:@[config]];
[self.navigationController pushViewController:requestController animated:YES];
Note: For more info on custom fields, check out our providers.
Configure the ticket screen of other SDK controllers
Use RequestUiConfiguration. Example:
Swift
let config = RequestUiConfiguration()
config.subject = "iOS Ticket"
config.tags = ["ios", "mobile"]
let requestListController = RequestUi.buildRequestList(with: [config])
self.navigationController?.pushViewController(requestListController, animated: true)
Objective-C
ZDKRequestUiConfiguration * config = [ZDKRequestUiConfiguration new];
config.subject = @"iOS Ticket";
config.tags = @[@"ios", @"mobile"];
UIViewController *requestController = [ZDKRequestUi buildRequestListWith:@[config]];
[self.navigationController pushViewController:requestController animated:YES];
Show the user's tickets
Use the buildRequestList()
method of the RequestUi. Example:
Swift
let requestListController = RequestUi.buildRequestList()
self.navigationController?.pushViewController(requestListController, animated: true)
Objective-C
UIViewController *requestListController = [ZDKRequestUi buildRequestList];
[self.navigationController pushViewController:requestListController animated:YES];
Show an existing ticket
Use the buildRequestUi(requestId: String?)
method of the RequestUi. Example:
Swift
let requestController = RequestUi.buildRequestUi(requestId: "<id>")
self.navigationController?.pushViewController(requestController, animated: true)
Objective-C
UIViewController *requestController = [ZDKRequestUi buildRequestUiWithRequestId:@"<id>"];
[self.navigationController pushViewController:requestController animated:YES];
Check for ticket updates
The Request List Screen shows a bullet next to a ticket to alert the user that the ticket has been updated. However, if you don't use the Request List Screen or if the user isn't checking their ticket list often, the user won't be aware of updates.
If you are using JWT authentication, the user's tickets submitted from other channels appear in their request list. They show as unread the first time the user views the request list, because the read/unread state is stored on the device. Viewing each request resets it to the read state, and any new updates after that cause the ticket to appear unread again.
Note: Identified users receive email notifications from Zendesk Support when their tickets are updated.
You can use an API provider to check if any of the user's tickets have been updated, and then alert the user based on the response. The ZDKRequestProvider has a getUpdatesForDevice()
method that checks for ticket updates. If any are found, you can alert the user elsewhere in your app and perhaps use the RequestController to show each ticket.
Example:
Swift
let requestProvider = ZDKRequestProvider()
requestProvider?.getUpdatesForDevice(callback: { (requestUpdates) in
if let requestUpdates = requestUpdates,
requestUpdates.hasUpdatedRequests() {
_ = requestUpdates.requestUpdates.flatMap { print("Request \($0.key) has \($0.value) updates") }
} else {
//Handle error / no updates
}
})
Objective-C
ZDKRequestProvider * provider = [ZDKRequestProvider new];
[provider getUpdatesForDeviceWithCallback:^(ZDKRequestUpdates * _Nullable requestUpdates) {
if (requestUpdates.hasUpdatedRequests) {
for (NSString * key in requestUpdates.requestUpdates) {
NSLog(@"Request %@ has %@ updates", key, requestUpdates.requestUpdates[key]);
}
} else {
//Handle error / no updates
}
}];
This method caches requestUpdates
for up to an hour. Any subsequent API call in that timeframe returns the cached object rather than making the API call.
Viewing a ticket removes it from the cached requestUpdates.requestsWithUpdates
object. The event occurs when the ticket's comments load in RequestController.
If you implement a custom UI, you can mark a ticket as seen by calling the following method with the ticket's ID and the number of comments that have been read (usually the total number of comments):
If you implemented any custom UI, you can mark a request as seen by calling the following method:
Swift
ZDKRequestProvider().markRequestAsRead("123", withCommentCount: 11)
Objective-C
ZDKRequestProvider * provider = [ZDKRequestProvider init];
[provider markRequestAsRead:@"123" withCommentCount:11];
If you've integrated push notifications for request updates but have implemented a custom request UI, then you can mark a ticket as unread when a push update is received:
ZDKRequestProvider().markRequestAsUnRead("123")
Objective-C
ZDKRequestProvider * provider = [ZDKRequestProvider init];
[provider markRequestAsUnread:@"123"];