Setting an identity (Required)
Setting an identity (Required)
To access your organization's help center or to submit tickets, a user of your app must have an identity so they can authenticate as a Zendesk Support user. You can set two types of identities for a user:
To determine which option is best for your organization, see Authentication decision in the SDK integration checklist.
On the Zendesk Support side, an administrator must enable the chosen option in the admin interface. If you're a Zendesk Support admin, see Registering the application in Zendesk Support in Zendesk help. Make sure the identity type you set in the SDK matches the type set in the admin interface. Mismatched identity types will cause the SDK network calls to fail with an error.
Note: The Zendesk Support SDK is intended for end users only. Authenticating with an agent email address returns a 403 authentication error. Be aware of this when testing your integration.
Setting an anonymous identity
Use this option if you don't know, or don't need to know, the details of the end user. For details, see Authentication decision in the SDK integration checklist. See How anonymous identities work in the mobile SDKs for more information about the lifecycle of an anonymous identity.
To set an anonymous identity
Swift
let identity = Identity.createAnonymous()
Zendesk.instance?.setIdentity(identity)
Objective-C
id<ZDKObjCIdentity> userIdentity = [[ZDKObjCAnonymous alloc] initWithName:nil email:nil];
[[ZDKClassicZendesk instance] setIdentity:userIdentity];
Adding identifying information to an anonymous identity
Even though the identity is anonymous, you can add the end user's name and email address to the identity. To decide whether or not to add this information, see User identification decision in the SDK integration checklist.
Note the following if you decide to add the information:
- Including an email address in the anonymous identity ensures that any new tickets are linked to the user profile in Zendesk Support with that email address.
- End users will only see the tickets they created on the mobile device with the Support SDK. This is because an anonymous identity is an untrusted one.
- Tickets created using an anonymous identity are flagged with warning to agents so that they know the end user wasn't signed in when they created the ticket.
Note: If you need to comply with the Federal Trade Commission's Children's Online Privacy Protection Act (COPPA), consider not providing any identifying information.
To add identifying information
-
Define the identity as follows:
Swift
let identity = Identity.createAnonymous(name: "John Bob", email: "[email protected]")
Zendesk.instance?.setIdentity(identity)
Objective-C
id<ZDKObjCIdentity> userIdentity = [[ZDKObjCAnonymous alloc] initWithName:@"John Bob"
email:@"[email protected]"];
[[ZDKClassicZendesk instance] setIdentity:userIdentity];
Setting a unique identity
If you want only trusted users to access your organization's help center or to submit tickets, your app must supply the SDK with a unique identity for the user. Zendesk must be able to use the identity to verify with your organization that the user is known and trusted. Your organization must provide Zendesk with a dedicated JWT endpoint to verify the identity.
After getting a unique identifier from your app, Zendesk will send it in a request to your dedicated JWT endpoint. Zendesk will expect a response containing a JWT token confirming that the user is known and trusted. The token must include name and email properties.
Here's the JWT authentication flow (enlarge):
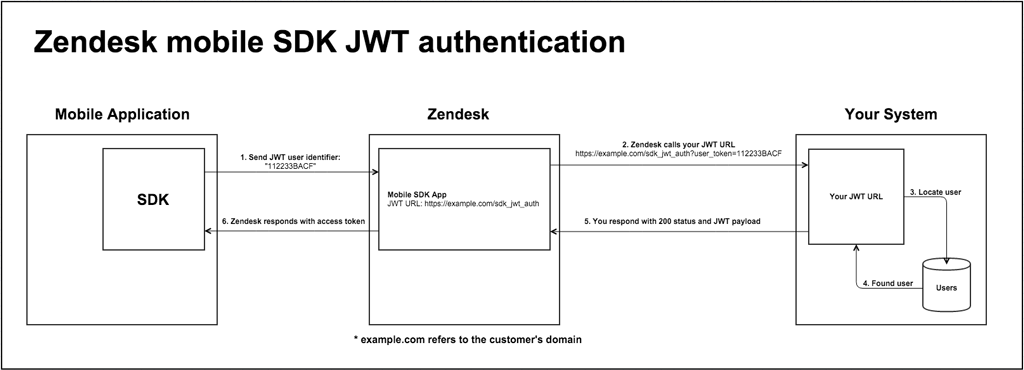
See Building a dedicated JWT endpoint for the Support SDK in Zendesk help.
Requirements
You must meet the following requirements to set this type of identity in the SDK:
-
Your organization built and maintains a dedicated JWT endpoint for the Support SDK. See Building a dedicated JWT endpoint for the Support SDK in Zendesk help.
-
Your organization has a user database and a way for the JWT endpoint to query it. Zendesk will send the unique identifier from your app to the JWT endpoint and wait for your system to look up the user and send a response that the user is known and trusted.
-
Your mobile app has access to the unique identifier used to look up the user in the database. The identifier can be whatever you want to use in the query.
A good example of a unique identifier is a user's access token that your app has after your user signs in. We don't recommend using something predictable like an email address or user id.
Setting unique identities
-
Add the following statement before you use the Zendesk SDK:
Swift
let identity = Identity.createJwt(token: "unique_id")
Zendesk.instance?.setIdentity(identity)
Objective-C
id<ZDKObjCIdentity> userIdentity = [[ZDKObjCJwt alloc] initWithToken:"unique_id"];
[[ZDKClassicZendesk instance] setIdentity:userIdentity];
The "unique_id" is different for each app user.
When to set an identity
An identity must be set after Zendesk.initialize
but before any action that interacts with the
Zendesk back-end (such as showing the help center or ticketing,
or using one of the API providers)
You can set it either before or after Support.initialize
, but attempting to
use the Support SDK without setting an identity will cause all network requests to fail.
Changing the identity
You can change the identity at any time by calling Zendesk.instance?.setIdentity
again with a
different identity object. Calling the method more than once with the same identity will have
no effect.
If using an anonymous identity, there are some important caveats to consider when changing identities:
-
Changing the identity will result in all data being wiped from the Support SDK's storage. Any open tickets will be lost and can never be accessed through the SDK again, even if you set the same identity again later.
-
Any change to an anonymous identity's details will be treated as a change of identity rather than updating the current identity. Consider the scenario where you are initially creating the identity using only the user's name:
let identity = Identity.createAnonymous(name: "John Bob", email: nil)
After finding out the user's email address later, you set the identity with the updated details
let identity = Identity.createAnonymous(name: "John Bob", email: "[email protected]")
The SDK treats this as a completely new user, and deletes any stored data (such as ticket IDs, which cannot be retrieved again).