Understanding the Support SDK
Understanding the Support SDK
Overview
If your organization uses Zendesk Support or Zendesk Guide for customer service, you can use the Support SDK for Android to embed the same customer service in an Android app.
The SDK provides the following activities for embedding customer service features in an app:
-
HelpCenterActivity - Lets the user access articles in your Zendesk Guide knowledge base and, optionally, submit a ticket. See Adding your help center
-
ViewArticleActivity - Lets the user view a specific help center article.
-
RequestActivity - Lets the user submit, view, and update tickets to your customer service team. See Adding a ticket form
-
RequestListActivity - Lets the user view a list of their tickets. See Adding a ticket list
The activities have their own user interface that you can customize for branding purposes. If using a Material Design theme, the SDK will inherit your primary colour, dark primary colour, and accent colour. This level of customization is suitable for most use cases. Example:
<style name="AppTheme" parent="Theme.MaterialComponents.Light.DarkActionBar">
<item name="colorPrimary">@color/my_color_primary</item>
<item name="colorPrimaryDark">@color/my_color_primary_dark</item>
<item name="colorAccent">@color/my_color_accent</item>
</style>
If the included UI doesn't meet the product requirements, you can build your own. The SDK includes API providers to connect a custom UI to Zendesk functionality. For example, the game Nibblers by Rovio uses a custom UI and the API providers:
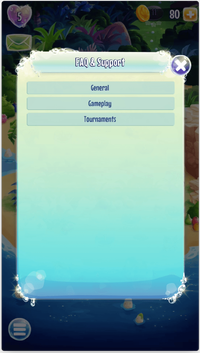
Dipping a toe
-
In a hurry? See Using the SDK in a nutshell
-
Browse this guide.
-
Ask questions in the Zendesk SDKs community.
-
Have a look at our sample apps on Github.
-
See the Support SDK Javadocs on Github.
Diving in
Ok, your organization has decided to use the Support SDK to embed customer service in your Android app.
-
Before you start, meet with product managers, the customer service team, and the mobile team to decide how to integrate the SDK in the app. Use the Zendesk SDK integration checklist for guidance.
-
After deciding how to integrate, make sure one of your Zendesk Support administrators has registered your app and turned on SDK features in the Support account.
See Registering the application in Zendesk Support in Zendesk help.
Make sure the features you want to use are covered in your Zendesk plan. See the breakdown of features and limitations per plan.
-
Add the Support SDK to your Android project (Required).
-
Initializing the Support SDK (Required).
-
Set a user identity for the SDK (Required).
The SDK uses the identity to access your Zendesk Support account as a user. -
Initialize the Support SDK in your app.
-
Add a customer service feature:
-
Customize the look and feel of the feature.
See Customize the look.
-
(Optional) Build your own UI in Android and then use the SDK's API providers to connect your UI to Zendesk functionality.
See API providers.
Common terms
-
Anonymous - One of two identity types supported by the Support SDK. (The other is JWT.) An anonymous user can be a user with no information associated with them, or a user with some identifying information such as a name and email. See Setting an identity.
-
API providers - A group of APIs included with the SDK that lets you build your own support features. See API providers.
-
Contact us, Contact Zendesk - Submit a ticket.
-
Help center - A self-service platform by Zendesk Guide consisting of a knowledge base and an online community. The SDK lets users access the knowledge base. Learn more on the product page.
-
Request - A request by an end user for support. The term is interchangeable with "ticket". The request is handled by your customer service team using Zendesk Support. Learn more on the product page.
-
Ticket - Same as a support request.
SDK fact sheet
Supported languages
The Support SDK supports 33 languages. For the full list, see Language codes for Zendesk supported languages in Zendesk help.
The SDK has full support for RTL (right-to-left) languages.
Accessibility
The Support SDK is fully accessible, with support for TalkBack and Switch Access.
Programming language
The Support SDK is written in Java. It does not include a dependency on Kotlin.
Android API compatibility
The current version of the Support SDK is compatible with Android API level 21 and higher.
Dependencies
As the support
module contains our UI code, and the support-providers
module doesn't, the
support
module has a larger list of dependencies.
Note: Please remember that using a higher version of one of the following dependency in your app may result in our Mobile SDKs not working as intended as the most recent version will be picked by Gradle. If you are using any of those dependencies, they should at least be in the same major as the version we are using.
'support'
+--- project :support-providers
+--- project :guide
+--- org.jetbrains.kotlin:kotlin-stdlib:1.9.24
+--- com.google.dagger:dagger-android:2.48.1
+--- androidx.multidex:multidex:2.0.0
+--- androidx.legacy:legacy-support-v4:1.0.0
+--- androidx.appcompat:appcompat:1.7.0
+--- androidx.recyclerview:recyclerview:1.1.0
+--- com.google.android.material:material:1.4.0
+--- androidx.cardview:cardview:1.0.0
+--- com.jakewharton:disklrucache:2.0.2
+--- com.squareup.picasso:picasso:2.8
\--- com.google.code.gson:gson:2.10.1
'support-providers'
+--- project :guide-providers
+--- org.jetbrains.kotlin:kotlin-stdlib-jdk8:1.6.10
+--- com.google.dagger:dagger:2.42
+--- androidx.annotation:annotation:1.3.0
+--- com.squareup.retrofit2:retrofit:2.9.0
\--- com.google.code.gson:gson:2.8.9
Footprint
The footprint is smaller if your app and the Support SDK share libraries.
Dependencies like support
contain UI elements and have a bigger footprint than the -providers
.
You can reduce the footprint with ProGuard.
Method count
The executable bytecode of Android apps is contained in Dalvik Executable (DEX) files. The Dalvik Executable specification limits the total number of methods that can be referenced within a single DEX file to 65,536. As your app grows, you could exceed this limit and start getting build errors.
The totals are calculated using the dexcount-gradle-plugin tool on a Zendesk sample application that hasn't been optimized with ProGuard.
Total methods in support-debug.aar: 3446 (5.26% used) Total fields in support-debug.aar: 1609 (2.46% used) Total classes in support-debug.aar: 774 (1.18% used) Methods remaining in support-debug.aar: 62089 Fields remaining in support-debug.aar: 63926 Classes remaining in support-debug.aar: 64761
The method count is much lower if you're only using the API providers:
Total methods in support-providers-debug.aar: 857 (1.31% used) Total fields in support-providers-debug.aar: 471 (0.72% used) Total classes in support-providers-debug.aar: 206 (0.31% used) Methods remaining in support-providers-debug.aar: 64678 Fields remaining in support-providers-debug.aar: 65064 Classes remaining in support-providers-debug.aar: 65329
For ways to avoid the 65K limit, see Enable Multidex for Apps with Over 64K Methods on the Android developer site.
The following is a breakdown of the method count with dependencies, for an empty app module (no classes) with a dependency on support
:
293 android
26 android.animation
6 android.app
30 android.content
3 android.content.pm
8 android.content.res
34 android.graphics
9 android.graphics.drawable
1 android.graphics.drawable.shapes
3 android.net
20 android.os
13 android.renderscript
33 android.text
2 android.text.format
1 android.text.method
7 android.text.style
1 android.text.util
3 android.util
56 android.view
5 android.view.animation
2 android.view.inputmethod
69 android.widget
107 androidx
31 androidx.appcompat
22 androidx.appcompat.app
9 androidx.appcompat.widget
4 androidx.cardview
4 androidx.cardview.widget
1 androidx.coordinatorlayout
1 androidx.coordinatorlayout.widget
11 androidx.core
3 androidx.core.app
2 androidx.core.content
2 androidx.core.graphics
2 androidx.core.graphics.drawable
2 androidx.core.util
2 androidx.core.view
1 androidx.core.view.animation
5 androidx.fragment
5 androidx.fragment.app
2 androidx.interpolator
2 androidx.interpolator.view
2 androidx.interpolator.view.animation
44 androidx.recyclerview
44 androidx.recyclerview.widget
4 androidx.swiperefreshlayout
4 androidx.swiperefreshlayout.widget
5 androidx.transition
87 com
29 com.google
22 com.google.android
22 com.google.android.material
3 com.google.android.material.appbar
4 com.google.android.material.bottomsheet
3 com.google.android.material.floatingactionbutton
7 com.google.android.material.snackbar
5 com.google.android.material.textfield
7 com.google.gson
1 com.google.gson.reflect
7 com.jakewharton
7 com.jakewharton.disklrucache
19 com.squareup
19 com.squareup.picasso
32 com.zendesk
5 com.zendesk.logger
2 com.zendesk.sdk
11 com.zendesk.service
14 com.zendesk.util
5 dagger
5 dagger.internal
175 java
12 java.io
60 java.lang
0 java.lang.annotation
2 java.text
101 java.util
11 java.util.concurrent
8 java.util.concurrent.atomic
2 java.util.logging
2 java.util.regex
1 javax
1 javax.inject
12 okhttp3
8 okio
13 org
13 org.xmlpull
13 org.xmlpull.v1
2745 zendesk
32 zendesk.belvedere
9 zendesk.configurations
31 zendesk.core
35 zendesk.messaging
13 zendesk.messaging.components
8 zendesk.messaging.components.bot
2638 zendesk.support
1613 zendesk.support.request
333 zendesk.support.requestlist
173 zendesk.support.suas
The following is a breakdown of the method count with dependencies, for an empty app module (no classes) with a dependency on support-providers
:
13 android
2 android.app
11 android.content
0 android.os
25 com
25 com.zendesk
5 com.zendesk.logger
2 com.zendesk.sdk
2 com.zendesk.sdk.providers
6 com.zendesk.service
12 com.zendesk.util
4 dagger
4 dagger.internal
73 java
5 java.io
32 java.lang
0 java.lang.annotation
4 java.text
32 java.util
1 java.util.concurrent
1 javax
1 javax.inject
2 okhttp3
1 retrofit2
738 zendesk
32 zendesk.core
706 zendesk.support