Adding your help center
The help center by Zendesk Guide provides your customers with a web-based, self-service knowledge base. You can use the Support SDK to show localized content in your help center, filter its contents to be more helpful, and use it as a jumping off point for creating a ticket.
The SDK provides the following two controllers to add your help center to your iOS app:
- HelpCenterOverviewController
- ViewArticleController
If you need to build your own UIs, the SDK also has an API provider that gives you access to your organization's help center. See Help Center provider in the API providers reference.
Topics covered in this section:
- Prerequisites
- UI overview
- Show the help center
- Filter articles by category
- Filter articles by section
- Filter articles by label
- Show a single article
- Configure the ticket screen
- Disable Contact Us and ticket creation
- Disable article voting
- Override device locale
Prerequisites
-
A Zendesk admin has activated help center in Zendesk Support. See Activating your help center in Zendesk help
-
One or more articles have been published in the knowledge base
-
a Zendesk admin has enabled Enable Guide for your iOS app in Zendesk Support. See Configuring the SDK in Zendesk Support in Zendesk help
UI overview
The default UI of the HelpCenterOverviewController looks as follows:
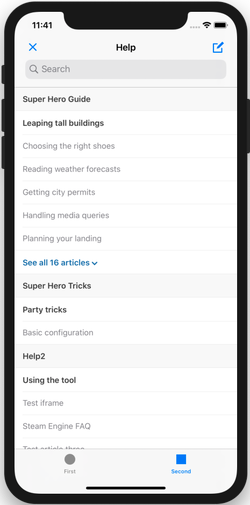
To change the look and feel of the UI, see Customize the look. The UI doesn't inherit any styling from the web-based version of your help center.
The UI is localized in 33 languages and selects the appropriate language based on the device locale. The locale must exist in your help center and in your Support account localization settings. The articles in your help center must be localized to view them in another language.
Tapping an article in the list shows the article content:
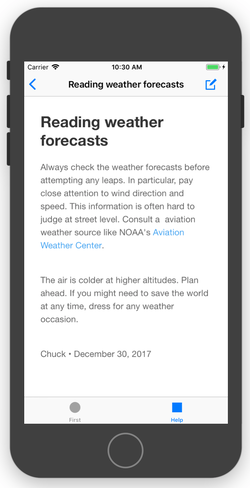
If the user can't find an answer in the help center, they have the option of tapping the pencil icon in the upper-right corner to open a ticket form:
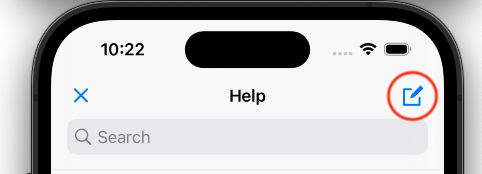
To configure the controller's ticket form, see Configure the ticket form of other SDK controllers.
You can disable the ticket form if you don't want it, or if you prefer adding a separate ticket form.
Show the help center
Use the buildHelpCenterOverviewUiWithConfigs
method of the HelpCenterUi. Example:
Swift
let helpCenter = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [])
self.navigationController?.pushViewController(helpCenter, animated: true)
Objective-C
UIViewController *helpCenter = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs:@[]];
[self.navigationController pushViewController:helpCenter animated:YES];
Note: Above a certain number of articles, the SDK starts to limit how many are fetched and displayed out of performance reasons. If this occurs, the recommended approach is to use the various filters on categories, sections, or labels that we provide to limit and control the amount of results. See Known issues.
Filter articles by category
Set the groupIds
and groupType
properties of the HelpCenterUiConfiguration. Example:
Swift
let hcConfig = HelpCenterUiConfiguration()
hcConfig.groupType = .category
hcConfig.groupIds = [1234, 5678]
let helpCenter = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [hcConfig])
self.navigationController?.pushViewController(helpCenter, animated: true)
Objective-C
ZDKHelpCenterUiConfiguration * hcConfig = [ZDKHelpCenterUiConfiguration new];
[hcConfig setGroupType:ZDKHelpCenterOverviewGroupTypeCategory];
[hcConfig setGroupIds:@[@1234, @5678]];
UIViewController *helpCenter = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs:@[hcConfig]];
[self.navigationController pushViewController:helpCenter animated:YES];
Filter articles by section
Set the groupIds
and groupType
properties of the HelpCenterUiConfiguration. Example:
Swift
let hcConfig = HelpCenterUiConfiguration()
hcConfig.groupType = .section
hcConfig.groupIds = [1234, 5678]
let helpCenter = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [hcConfig])
self.navigationController?.pushViewController(helpCenter, animated: true)
Objective-C
ZDKHelpCenterUiConfiguration * hcConfig = [ZDKHelpCenterUiConfiguration new];
[hcConfig setGroupType:ZDKHelpCenterOverviewGroupTypeSection];
[hcConfig setGroupIds:@[@1234, @5678]];
UIViewController *helpCenter = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs:@[hcConfig]];
[self.navigationController pushViewController:helpCenter animated:YES];
Filter articles by label
Set the labels
property of the HelpCenterUiConfiguration. Example:
Swift
let hcConfig = HelpCenterUiConfiguration()
hcConfig.labels = ["ios", "xcode"]
let helpCenter = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [hcConfig])
self.navigationController?.pushViewController(helpCenter, animated: true)
Objective-C
ZDKHelpCenterUiConfiguration * hcConfig = [ZDKHelpCenterUiConfiguration new];
[hcConfig setLabels:@[@"ios", @"xcode"]];
UIViewController *helpCenter = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs:@[configs]];
[self.navigationController pushViewController:helpCenter animated:YES];
Note: The labels are applied to the query by AND rather than OR. Returned content must match all specified labels.
Show a single article
To open a specific article, get the article's id, then use buildHelpCenterArticle.
Swift
let articleController = HelpCenterUi.buildHelpCenterArticleUi(withArticleId: 123, andConfigs: [])
self.navigationController?.pushViewController(articleController, animated: true)
Objective-C
UIViewController *articleController = [ZDKHelpCenterUi buildHelpCenterArticleUiWithArticleId:123 andConfigs:@[]];
[self.navigationController pushViewController:articleController animated:YES];
Configure the ticket screen
You can configure the ticketing screen of the HelpCenterOverviewController so that each new ticket has a common subject, common tags, or common file attachments. You can also set common values for one or more custom ticket fields, or set the custom ticket form to use in the agent interface in Zendesk Support.
First, configure the ticket screen with RequestUiConfiguration. See config in adding tickets.
Second, pass the Configuration object to the andConfigs
parameter of the ZDKRequestUi functions.
Example:
Swift
let requestConfig = RequestUiConfiguration()
requestConfig.subject = "iOS Ticket"
let helpCenter = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [requestConfig])
self.navigationController?.pushViewController(helpCenter, animated: true)
Objective-C
ZDKRequestUiConfiguration * requestConfig = [ZDKRequestUiConfiguration new];
requestConfig.subject = @"iOS Ticket";
UIViewController *helpCenter = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs:@[requestConfig]];
[self.navigationController pushViewController:helpCenter animated:YES];
Disable Contact Us button and ticket creation
The help center has a Contact Us button on the navigation bar that you can use to create a ticket.
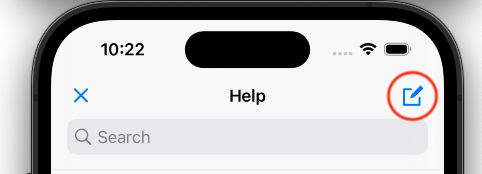
The button is visible by default in both the help center article list view and article view. You can hide the button in one or both places. This is done using the HelpCenterUiConfiguration and the ArticleUiConfiguration.
Disable ticket creation in the help center
On the help center screen, the ticket creation button appears in two places:
- The navbar on the main screen
- The search result screen when there are no search results
HelpCenterUiConfiguration has a boolean property for configuring the visibility of the ticket creation button in the two locations. It is false by default. You can customize the value as required.
Example:
Swift
func presentHelpCenter() {
let helpCenterUiConfig = HelpCenterUiConfiguration()
helpCenterUiConfig.showContactOptions = false
let articleUiConfig = ArticleUiConfiguration()
articleUiConfig.showContactOptions = false // hide in article screen
let helpCenterViewController = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [helpCenterUiConfig, articleUiConfig])
self.navigationController?.pushViewController(helpCenterViewController, animated: true)
}
Objective-C
-(void) presentHelpCenter {
ZDKHelpCenterUiConfiguration* helpCenterUiConfig = [ZDKHelpCenterUiConfiguration new];
[helpCenterUiConfig setShowContactOptions: NO];
ZDKArticleUiConfiguration* articleUiConfig = [ZDKArticleUiConfiguration new];
[articleUiConfig setShowContactOptions: NO];
UIViewController* controller = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs: @[helpCenterUiConfig, articleUiConfig]];
[self.navigationController pushViewController:controller animated:YES];
}
Disable ticket creation in the article view
ArticleUiConfiguration has a boolean property for configuring the visibility of the ticket creation button on the article screen. It is false by default. You can customize the value as required.
Example:
Swift
func presentArticle() {
let articleUiConfig = ArticleUiConfiguration()
articleUiConfig.showContactOptions = false
let articleViewController = HelpCenterUi.buildHelpCenterArticleUi(withArticleId: 123, andConfigs: [articleUiConfig])
self.navigationController?.pushViewController(articleViewController, animated: true)
}
Objective-C
-(void) presentArticle {
ZDKArticleUiConfiguration* articleConfig = [ZDKArticleUiConfiguration new];
[articleConfig setShowContactOptions: NO];
UIViewController* controller = [ZDKHelpCenterUi buildHelpCenterArticleUiWithArticleId:123 andConfigs:@[articleConfig]];
[self.navigationController pushViewController:controller animated:YES];
}
Disable ticket creation after an empty search result
Set showContactOptions
property of the HelpCenterUiConfiguration. Example:
Swift
func presentHelpCenter() {
let hcConfig = HelpCenterUiConfiguration()
hcConfig.showContactOptions = false
let helpCenter = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [hcConfig])
self.navigationController?.pushViewController(helpCenter, animated: true)
}
Objective-C
- (void) presentHelpCenter {
ZDKHelpCenterUiConfiguration * hcConfig = [ZDKHelpCenterUiConfiguration new];
[hcConfig setShowContactOptions:NO];
UIViewController* helpCenter = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs: @[hcConfig]];
[self.navigationController pushViewController:helpCenter animated:YES];
}
Hide the contact button in the My Tickets page
You can hide the Contact Us button on your My Tickets page but setting allowRequestCreation
to false.
For example:
let requestListConfig = RequestListUIConfiguration()
requestListConfig.allowRequestCreation = false
let requestList = RequestUi.buildRequestList(with: [requestListConfig])
navigationController.pushViewController(requestList, animated: true)
Disable article voting
The help center article voting feature in the Support SDK can be enabled and disabled by a Zendesk admin in Zendesk Support. It is enabled by default. See Configuring the SDK in Zendesk Support in Zendesk help.
This article voting is entirely separate to resolutions and rejections in Answer Bot.
Using Support SDK with Answer Bot SDK
Note: Zendesk has renamed our bot capabilities. Answer Bot is now AI agents.
Answer Bot by Zendesk, provides a powerful mechanism for deflecting tickets before they reach your agents.
If you have Answer Bot 2.0.0 and Support 5.0.0 (or above) present in your app, you can configure the contact button in HelpCenterOverviewController
and ViewArticleController
to open the Answer Bot Engine. If you do not provide any engine, the user will be directed to ticket creation by default.
Swift
func presentHelpCenter() {
do {
let answerBotEngine = try AnswerBotEngine.engine()
let helpCenterUiConfig = HelpCenterUiConfiguration()
helpCenterUiConfig.engines = [answerBotEngine]
let articleUiConfig = ArticleUiConfiguration()
articleUiConfig.engines = [answerBotEngine]
let helpCenterViewController = HelpCenterUi.buildHelpCenterOverviewUi(withConfigs: [helpCenterUiConfig, articleUiConfig])
self.navigationController?.pushViewController(helpCenterViewController, animated: true)
} catch {
// do something with error
}
}
Objective-C
-(void) presentHelpCenter {
NSError *error = nil;
ZDKAnswerBotEngine *abEngine = [ZDKAnswerBotEngine engineAndReturnError:nil];
NSArray *engines = @[(id <ZDKEngine>)abEngine];
ZDKHelpCenterUiConfiguration* helpCenterUiConfig = [ZDKHelpCenterUiConfiguration new];
helpCenterUiConfig.objcEngines = engines;
ZDKArticleUiConfiguration* articleUiConfig = [ZDKArticleUiConfiguration new];
articleUiConfig.objcEngines = engines;
UIViewController* controller = [ZDKHelpCenterUi buildHelpCenterOverviewUiWithConfigs: @[helpCenterUiConfig, articleUiConfig]];
[self.navigationController pushViewController:controller animated:YES];
}
Overriding device locale
If you want to manually specify what language to use when fetching help center content you can do so with:
Swift
Support.instance().helpCenterLocaleOverride = "<IETF language tag>"
Objective-C
[ZDKSupport instance].helpCenterLocaleOverride = @"<IETF language tag>";
If you're only using the API providers and building the UI yourself:
Swift
Support.instance().helpCenterLocaleOverride = "<IETF language tag>"
Objective-C
[ZDKSupport instance].helpCenterLocaleOverride = @"<IETF language tag>";
This applies to all help center content fetched with the UI and providers, meaning that only the articles, sections, and categories from this locale are fetched. This does not update the mobile UI elements.
The language tag must be all lowercase in order to work.
The override ignores the device locale and the languages configured in the Zendesk Guide admin console. Overriding with
an unsupported Locale
or no content for that locale results in a "Unable to connect" banner.